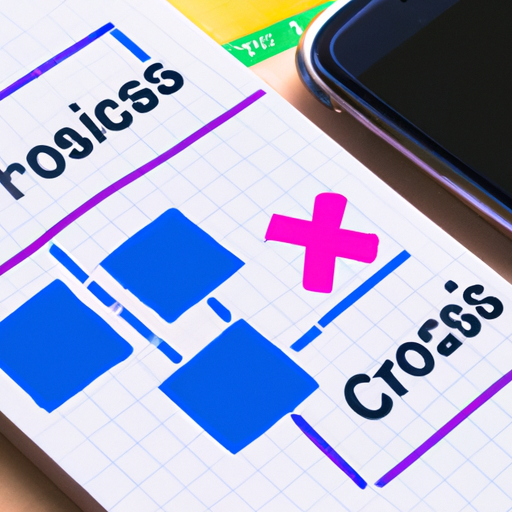
Building Cross-platform Mobile Apps with React Native and Typescript
Introduction
In today's digital age, mobile applications have become an essential part of our lives. With the increasing demand for mobile apps, developers are constantly looking for efficient ways to build high-quality apps that can run on multiple platforms. React Native, a popular JavaScript framework, and Typescript, a statically typed superset of JavaScript, have emerged as powerful tools for building cross-platform mobile apps. In this blog post, we will explore how to use React Native and Typescript to build cross-platform mobile apps.
Body
1. What is React Native?
React Native is an open-source framework developed by Facebook that allows developers to build mobile apps using JavaScript. It uses the same design principles as React, a popular JavaScript library for building user interfaces. React Native allows developers to write code once and deploy it on multiple platforms, such as iOS and Android. It provides a set of pre-built components that can be used to create a native-like user interface.
2. What is Typescript?
Typescript is a statically typed superset of JavaScript that adds optional static typing to the language. It provides features such as type checking, interfaces, and classes, which help developers catch errors early in the development process. Typescript code is transpiled into plain JavaScript, which can be run on any JavaScript runtime.
3. Why use React Native with Typescript?
Using React Native with Typescript offers several advantages for building cross-platform mobile apps:
- Type safety: Typescript provides static typing, which helps catch errors at compile-time and improves code quality.
- Code reusability: React Native allows developers to write code once and deploy it on multiple platforms. Typescript enhances code reusability by providing type definitions and interfaces.
- Tooling support: Typescript has excellent tooling support, including code editors with autocompletion, refactoring, and debugging capabilities.
- Community support: React Native and Typescript both have large and active communities, which means developers can find plenty of resources, tutorials, and libraries to help them build their apps.
4. Setting up a React Native project with Typescript
To start building a cross-platform mobile app with React Native and Typescript, you need to set up a development environment. Here are the steps:
- Install Node.js: React Native requires Node.js to run. You can download and install Node.js from the official website.
- Install React Native CLI: React Native provides a command-line interface (CLI) for creating and managing projects. You can install it globally using the following command:
npm install -g react-native-cli
- Create a new React Native project: Once you have installed the CLI, you can create a new React Native project using the following command:
react-native init MyApp --template react-native-template-typescript
- Start the development server: Navigate to the project directory and start the development server using the following command:
cd MyApp
npm start
- Run the app on a simulator or device: To run the app on a simulator or device, you need to have Xcode (for iOS) or Android Studio (for Android) installed. You can then run the app using the following command:
react-native run-ios
5. Writing React Native components with Typescript
React Native components are the building blocks of a mobile app's user interface. With Typescript, you can enhance the development experience by adding type annotations and interfaces to your components. Here's an example of a simple React Native component written in Typescript:
import React from 'react';
import { View, Text, StyleSheet } from 'react-native';
interface Props {
name: string;
}
const HelloWorld: React.FC = ({ name }) => {
return (
Hello, {name}!
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
});
export default HelloWorld;
In this example, we define a functional component called HelloWorld that takes a prop called name of type string. The component renders a View with a Text component inside it, displaying a greeting message.
6. Building platform-specific UI
While React Native allows you to write code once and deploy it on multiple platforms, there may be cases where you need to customize the UI for each platform. React Native provides a Platform module that allows you to conditionally render platform-specific UI components. Here's an example:
import React from 'react';
import { View, Text, StyleSheet, Platform } from 'react-native';
const HelloWorld: React.FC = () => {
return (
Hello, World!
{Platform.OS === 'ios' && Running on iOS }
{Platform.OS === 'android' && Running on Android }
);
};
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
},
text: {
fontSize: 20,
fontWeight: 'bold',
},
});
export default HelloWorld;
In this example, we use the Platform.OS property to conditionally render platform-specific Text components.
Conclusion
React Native and Typescript provide a powerful combination for building cross-platform mobile apps. With React Native, you can write code once and deploy it on multiple platforms, while Typescript adds type safety and tooling support to the development process. By following the steps outlined in this blog post, you can start building your own cross-platform mobile apps with React Native and Typescript.
References:
- React Native documentation: https://reactnative.dev/docs/getting-started
- Typescript documentation: https://www.typescriptlang.org/docs/
- React Native Typescript Starter: https://github.com/react-native-community/react-native-template-typescript
'FrontEnd' 카테고리의 다른 글
React Native 0.72 변경점 (0) | 2023.07.14 |
---|---|
React Native를 사용하여 타입스크립트와 React Query 적용하기 (0) | 2023.07.12 |
React Native 중첩 네비게이션과 Typescript의 깊은 탐구 (0) | 2023.07.11 |
React Native와 함께 사용하는 TypeScript의 이점 및 사용 방법 (0) | 2023.07.11 |
React Native에서 Text Input 컴포넌트 label 색상 변경하는 방법 (0) | 2023.07.11 |